Seam Faces makes JSF entity converters a breeze
One of the first Seam 3 Modules to appear is the Seam Faces module which provides additional functionality to JSF. While there aren’t many pain points left in JSF, one of the biggest is the issue of data converters for entity objects. This article will take a look at how Seam Faces takes the pain out of writing JSF converters.
Download
Download the Seam Faces Demo Source for Maven
Typically, JSF converters come in two different types, the first converts strings to other strings, numbers or dates and vice-versa. Examples might be dates, formatted phone numbers or social security numbers. These converter are usually isolated and rely on internal logic defined in the converter.
The second kind, which is far more problematic, involves using information outside the converter to perform the conversion. The simplest case being an entity lookup which is represented in the view layer by the primary key value and upon posting back to the server, converted to the entity based on that id. These converters require some mechanism to get the entity from the database based on the Id. JSF doesn’t allow any kind of resource injection in converters so it was always a problem. Seam 2 used a s:convertEntity
tag just for the purpose of loading the entity from the database. With Seam 3 however, you’ll be able to write your own converter using standard JSF rather than using specialized tags.
The Seam 3 module provides features that augment the existing JSF feature set and provides among other things, converters that can have beans injected to them like they are CDI beans. With this, we can implement converters that take the Id and load the entity from the database.
Here’s some quick demo code to demonstrate the process. The application uses the jee6-sandbox Knappsack archetype and requires either Glassfish or JBoss AS 6 to run.
I created a new project and in the DataFactory.java
class I added a producer method to return a list of teachers.
@Produces @Named("teachers") @ApplicationScoped public List<Teacher> getTeachers() { List<Teacher> teachers = entityManager.createQuery( "select t from Teacher t") .getResultList(); return teachers; }
This makes an application scoped list of teachers available in our application, where they will be used to provide a lookup list.
Now we’ll create a backing bean that has a teacher attribute that we want to set via the lookup list.
package org.knappsack.demo.seam.seamfaces.bean; import javax.enterprise.context.RequestScoped; import javax.inject.Named; import org.knappsack.demo.seam.seamfaces.model.Teacher; @Named @RequestScoped public class PageBean { private Teacher selected; public Teacher getSelected() { return selected; } public void setSelected(Teacher selected) { this.selected = selected; } }
No we’ll write our converter which implements the javax.faces.convert.Converter
interface.
@SessionScoped @FacesConverter("convert.teacher") public class TeacherConverter implements Converter,Serializable { @Inject @DataRepository private EntityManager entityManager; @Override public Object getAsObject(FacesContext context, UIComponent component, String value) { Long id = Long.valueOf(value); return entityManager.find(Teacher.class, id); } @Override public String getAsString(FacesContext context, UIComponent component, Object value) { return ((Teacher)value).getId().toString(); } }
The @FacesConverter
annotation marks this class as a converter when it is processed by CDI and gives it a converter Id for use in a JSF page.
To convert the object to text, we just return the id
of the object. To convert from text to an object (a Teacher
instance) we load the instance from the database using the injected entity manager.
To use this converter we just add this content of the home.xhtml
page :
<ui:define name="content"> <h:form id="form"> <h:outputText value="Selected : #{pageBean.selected.name}" id="msg" /> <br /> <h:selectOneListbox value="#{pageBean.selected}" id="selector" converter="convert.teacher"> <f:selectItems value="#{teachers}" id="items" var="v_teacher" itemLabel="#{v_teacher.name}" /> </h:selectOneListbox> <h:commandButton value="Update" action="update" /> </h:form> </ui:define>
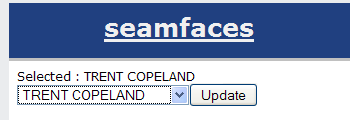
This page presents a list of teachers in which you can select one, click the update button and the selected teacher message at the top will change. The the only additional code we have is the converter which is a very simple class to write and can be re-used everywhere we have a teacher entity by just specifying the converter name. You could even create a generic entity converter to be used for all your entities.
Injection is not just limited to entity managers, we can inject any kind of bean in there so our options are limitless. One good use case is the injection and re-use of application scoped lists of cached data instead of re-hitting the database all the time.
Download the Seam Faces Demo Source for Maven, unzip it and see the readme.txt
file for deployment instructions.
7 thoughts on “Seam Faces makes JSF entity converters a breeze”
Comments are closed.
It is very disapointing that JSF 2.0 doesn’t support this out of the box.
I’m having trouble getting Seam 3 working, probably because it is still alpha code.
I’m looking into the alternative here:
http://dominikdorn.com/2010/04/cdi-weld-manual-bean-lookup/
Also, when I download the zip file for this project and try to build it with Maven (mvn clean package) I get the following:
[INFO] Failed to resolve artifact.
GroupId: org.jboss.spec
ArtifactId: jboss-javaee-6.0
Version: 1.0.0.Beta4
Reason: Unable to download the artifact from any repository
org.jboss.spec:jboss-javaee-6.0:pom:1.0.0.Beta4
from the specified remote repositories:
central (http://repo1.maven.org/maven2),
oss.sonatype.org/jboss-snapshots (http://oss.sonatype.org/content/repositories/jboss-snapshots)
Ryan, yes, I agree, it is a shame that this isn’t part of the spec. I can see why though to a degree, CDI is new and JSF shouldn’t have a dependency on it. However, I’m fairly confident that this kind of thing will make it into the next version in some shape or form.
Thanks for the heads up on the download problem. One downside of Maven is if you have the jar locally, it won’t tell you if there is a problem.
I’ll take a look and get back to you.
Cheers,
Andy
Ok, I found the problem, in the pom.xml file, change the JBoss repository URL to point to :
http://repository.jboss.org/nexus/content/groups/public-jboss/
I need to update the Knappsack archetypes to use that repository instead. I’ll also fix it in the downloadable project source.
Cheers,
Andy Gibson
Andy,
Thanks for looking into it. However, I grabbed your latest version and I still get the same error. I finally got it to build by upgrading from maven 2.2.1 to maven 3.0. Is it possible that this project requires maven 3? I thought I saw on the Seam site that seam requires maven 3.
Ryan
Hey Ryan,
Umm, interesting, yes, Seam 3 does require maven 3, but I wouldn’t have thought that it would make my project dependent on Maven 3. I personally use Maven 3, but I’m wondering if some of the pom syntax in the Seam 3 pom requires Maven 3….I guess that might be the case. I’ll have a look at it and see if that is the case.
Cheers,
Andy Gibson
Since this article is about making entity converters a breeze it is probably worth mentioning in your article that you don’t even have to specify a converter name in your facelets pages if you use the forClass attribute instead of the value attribute in the @FacesConverter annotation.